APIを使用してデータの取得、変更をするにCRUDがありますが、pythonのrequestsを使用してこれらを試してみます。
非常に簡単に言うとGETはサーバーからデータを取得、POSTはこちらからサーバーへデータを送る、UPDATEは削除、DELETEは削除です。文字通りですね。
この記事はUdemyのpython講座を参照しています。
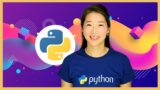
使用するサイト
pixelaを使用します。
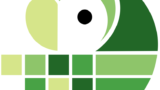
githubのように日々の習慣を入力すると、色の濃淡でどれだけ頑張ったかが一目でわかります。無料APIサービスです。
使い方の欄からその通りに進めていけば、実際にグラフを作成することができます。今回はグラフを作成したところから、進めていきたいと思います。
GET-データの取得
まずは作成したグラフを確認しておきましょう。APIドキュメントはこちら
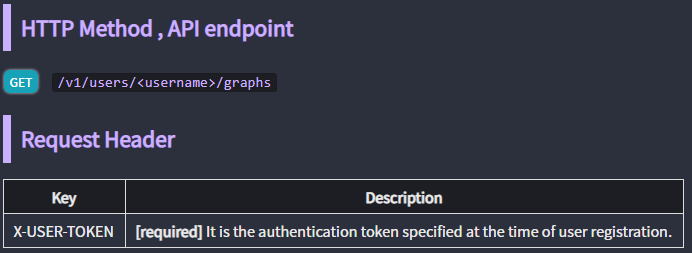
endpointというのがこのAPIを利用するためのURLです。<>の中身はユーザーに応じて変更、Request Headerの欄には与えられるパラメータが記載されています。X-USER-TOKENは[required]なので必須ということですね。
コード内のresponse.text
はコードが問題なく完了できているかどうかを見ています。
import requests
pixela_endpoint = "https://pixe.la/v1/users"
get_endpoint = "{}/{}/graphs".format(pixela_endpoint, USERNAME)
headers = {
"X-USER-TOKEN": TOKEN,
}
response = requests.get(get_endpoint, headers=headers)
print(response.text)
# {"graphs":[{"id":"graph1","name":"Cycling Graph","unit":"Km","type":"float","color":"ajisai","timezone":"","purgeCacheURLs":null,"selfSufficient":"none","isSecret":false,"publishOptionalData":false}]}
作成したグラフの情報を取得することができました。APIによってはgetメソッド内でparamを指定し、特定の情報を取得することも多いです。
POST-データを作成
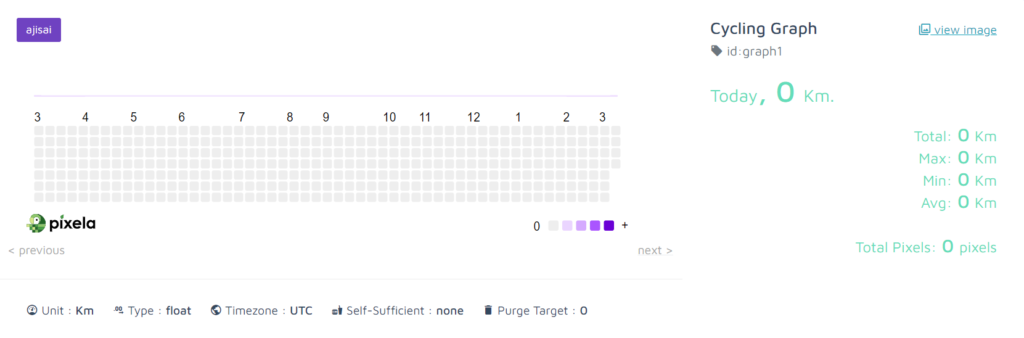
こちらが作成したグラフを可視化したものです。ここにPOSTを用いてデータを格納します。
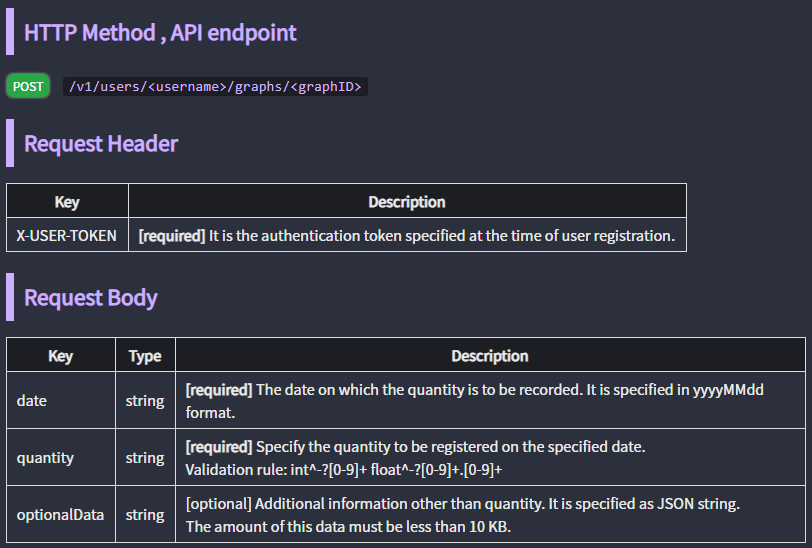
先ほどのHeaderに加え、Bodyにも[required]があることがわかります。日付と、どれだけ行ったかの量ですね。
コードにすると
import requests
from datetime import datetime
pixela_endpoint = "https://pixe.la/v1/users"
record_endpoint = "{}/{}/graphs/{}".format(pixela_endpoint, USERNAME, GRAPH_ID)
today = datetime.now()
headers = {
"X-USER-TOKEN": TOKEN,
}
record_config = {
"date": today.strftime("%Y%m%d"),
"quantity": input("How many kilometers did you cycle today? :"),
}
response = requests.post(url=record_endpoint, json=record_config, headers=headers)
print(response.text)
header情報と異なりbody情報はjson引数に与えています。
実行すると(15.8km走ったということにしました)
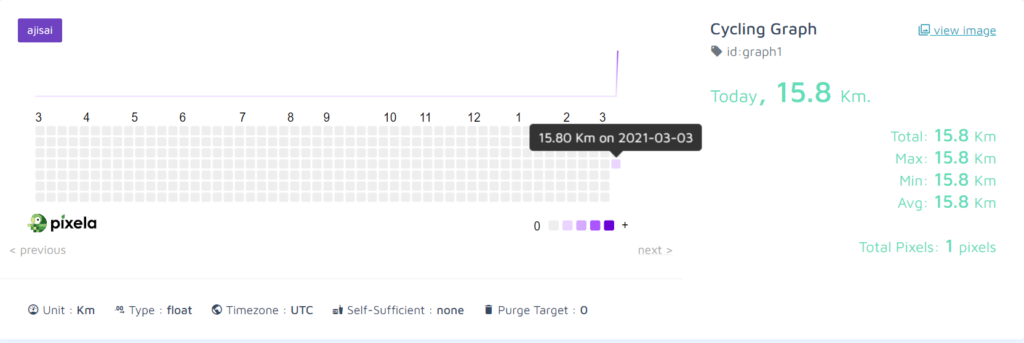
無事データが入りました!
UPDATE-データの更新
データの更新のために何日分かのデータを作成しておきました。
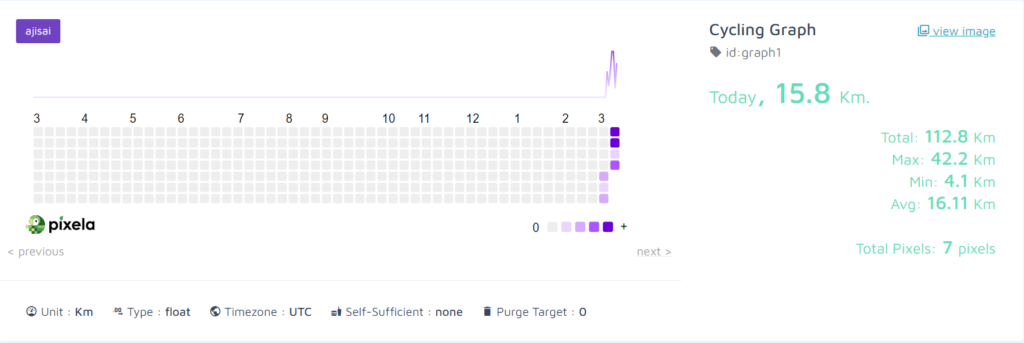
データを作成したのちに、昨日のデータ(4.1km)が少なすぎるのでずるして増やそうと思います。
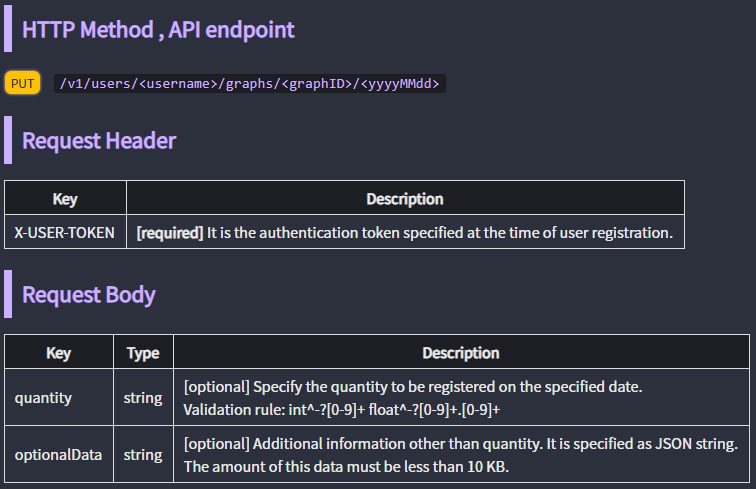
変更したい日付をendpointに加え、その日のquantityを与えればよいようです。
update_day = datetime(year=2021, month=3, day=2).strftime("%Y%m%d")
update_endpoint = "{}/{}/graphs/{}/{}".format(pixela_endpoint, USERNAME, graphID, update_day)
update_config = {
"quantity": "10.2",
}
response = requests.put(url=update_endpoint, json=update_config, headers=headers)
print(response.text)
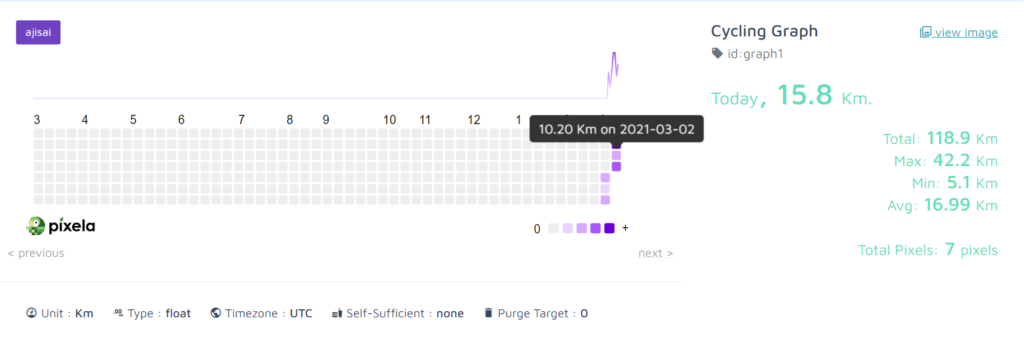
昨日の走った距離が変更されました。
DELETE-データの削除
先ほどずるして更新した距離ですが、思い直して、いっそずるして増やすくらいなら消してしまうことにします。
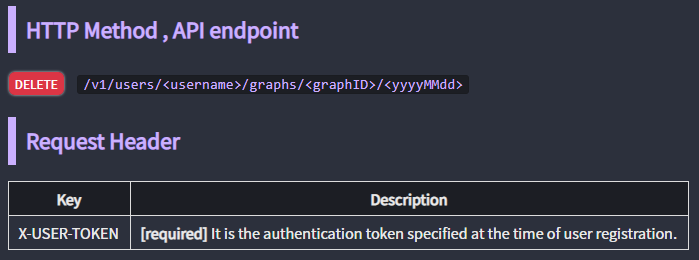
endpointに日付を入れて、TOKEN情報を入れればその日のデータを削除できるようです。
delete_day = datetime(year=2021, month=3, day=2).strftime("%Y%m%d")
delete_endpoint = "{}/{}/graphs/{}/{}".format(pixela_endpoint, USERNAME, graphID, delete_day)
response = requests.delete(url=delete_endpoint, headers=headers)
print(response.text)
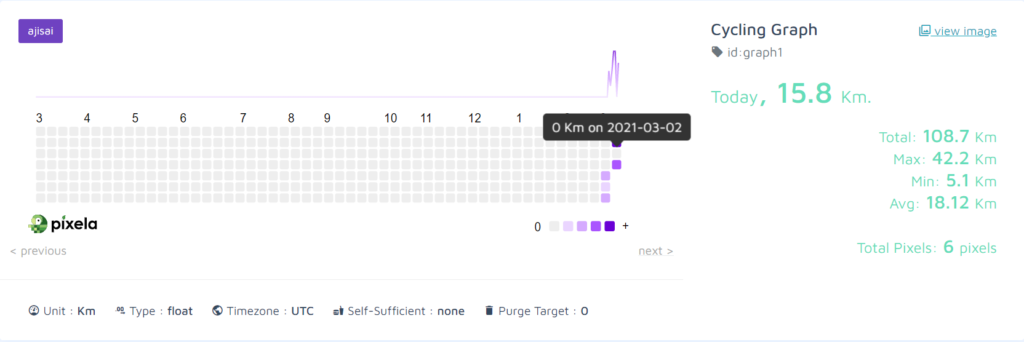
削除できました。
コメント